This is a diagram that I have used for this lab.
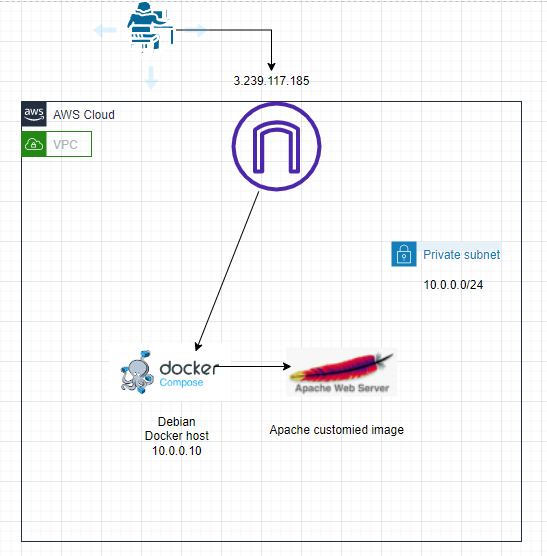
I have explained how to build a customized Docker image using Docker compose on-prem (https://tungle.ca/?p=2486). In this post, I will build a customized Docker image using Docker compose on AWS, then deploy WordPress via this docker container.
+ Create a new Debian Linux instance on AWS. Then, SSH to the instance and check Debian’s host version.
lsb_release -a
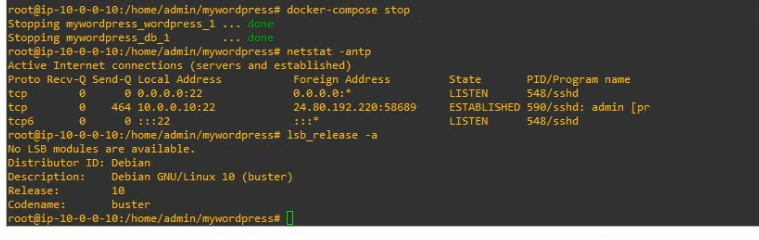
+ Create an index.php file with your customized information.
nano index.php
<?php
$yourname = "Tung Blog!";
$yourstudentnumber = "A123456789";
$image="tung.jpg"; // this must be included and uploaded as yourpic.jpg in your docker image (Dockerfile)
$uname=php_uname();
$all_your_output = <<<HTML
<html>
<head>
<meta charset="utf-8"/>
<title>$yourname - $yourstudentnumber</title>
</head>
<body>
<h1>$yourname - $yourstudentnumber</h1>
<img src="/$image">
<div>$uname</div>
</body>
<html>
HTML;
echo $all_your_output;
?>
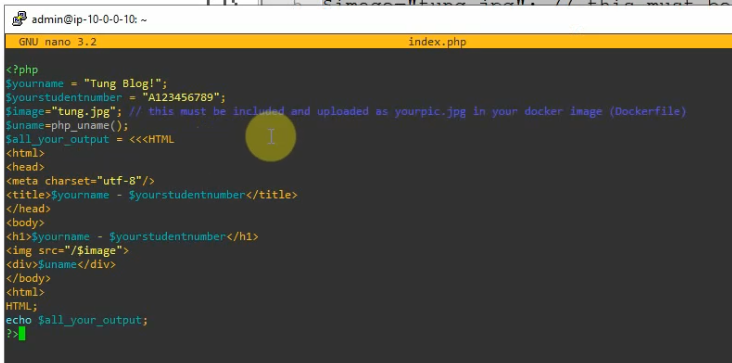
Download a free .jpg image from the Internet and change it to tung.jpg

This tells Docker to:
– Build an image starting with the Debian 10 image.
– Label the container with your email address.
– Install Apache web service and PHP module.
– Remove the default index.html on the Apache web server document root directory.
– Copy a new index.php file and your customized image to the Apache document root directory on the docker container.
– Run the command hostname and apachectl -DFOREGROUND runs in the foreground.
– Image to describe that the container is listening on port 80.
nano Dockerfile
root@docker01:~# cat Dockerfile
FROM debian:10
LABEL maintainer="xyz@my.bcit.ca"
#COPY index.php /usr/local/apache2/htdocs
#COPY index.php /var/www/html
#RUN apt-get update && apt-get -y install apache2
RUN apt update && apt -y install apt-utils systemd && apt-get -y install libapache2-mod-php
RUN rm /var/www/html/index.html
COPY index.php /var/www/html
COPY tung.jpg /var/www/html
#CMD apachectl -D FOREGROUND
CMD hostname TungA012345678 && apachectl -D FOREGROUND
EXPOSE 80
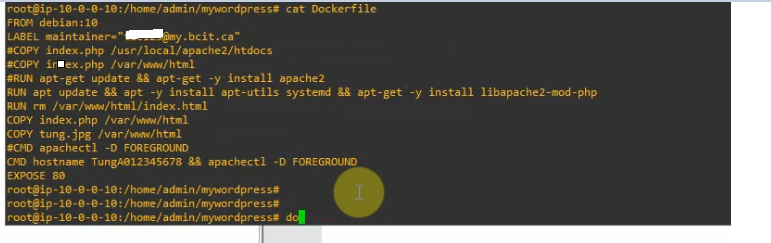
+ Build your app with Docker Compose.
docker build -t tung-a0123456789 .
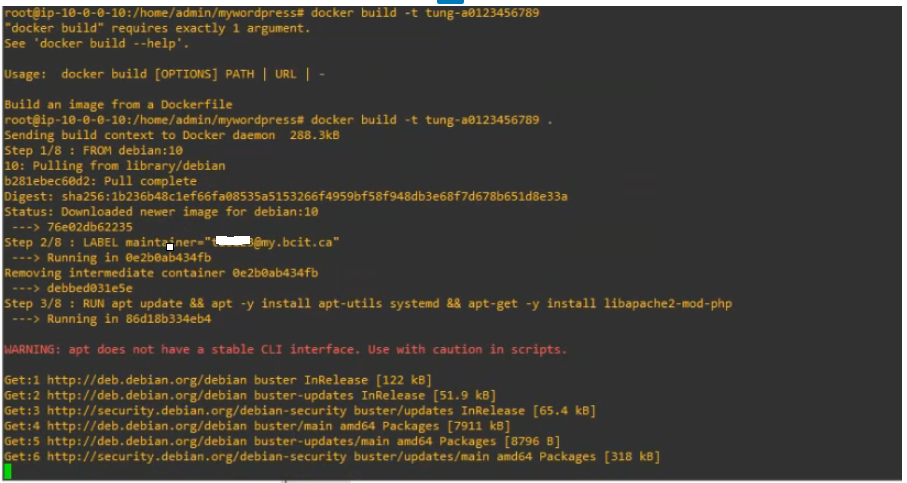
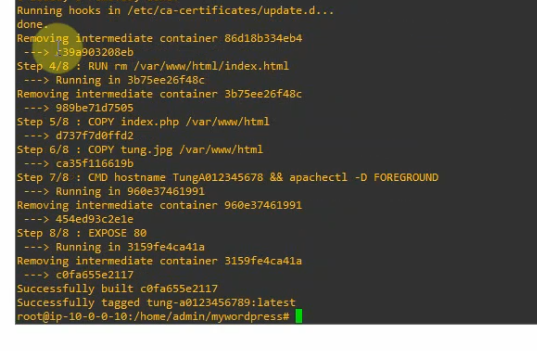
Run your app with Docker compose.
docker run -d -p 80:80 --cap-add sys_admin -dit tung-a0123456789
---
-- -d starts docker in daemon mode, in the foreground.
-- -d p 80:80 listening the port 80 on docker container
-- -cap-add sys_admin: basically root access to the host.
-- -dit: it is used for getting access to terminal inside a docker container. In this example is tung-a0123456789.

Check that port 80 is running on the docker container.
netstat -antp | grep 80

+ Check your application is running on a Docker container.
docker container ps -a

Connect to the Apache website with the PHP module on the docker container (http://3.239.117.185)
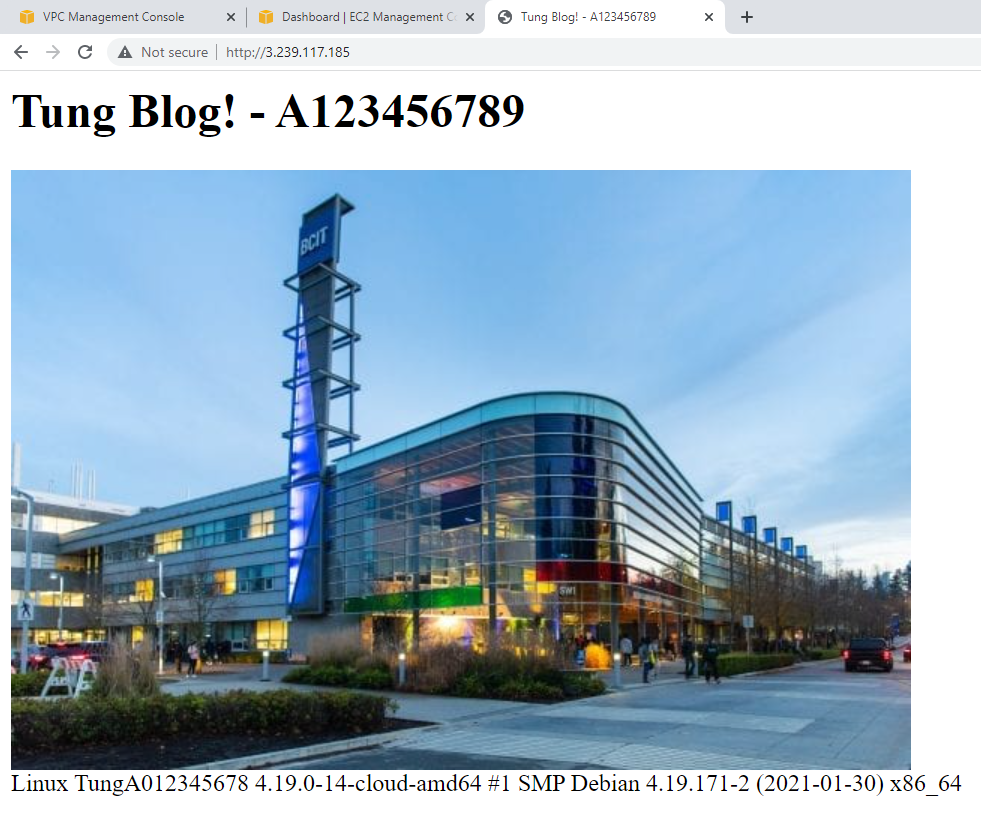
A few commands to use for checking the docker container.
docker ps -a
docker images
docker container ps -a
docker stop "Container ID"
docker rm "Container ID"
docker image rm "ImageID"
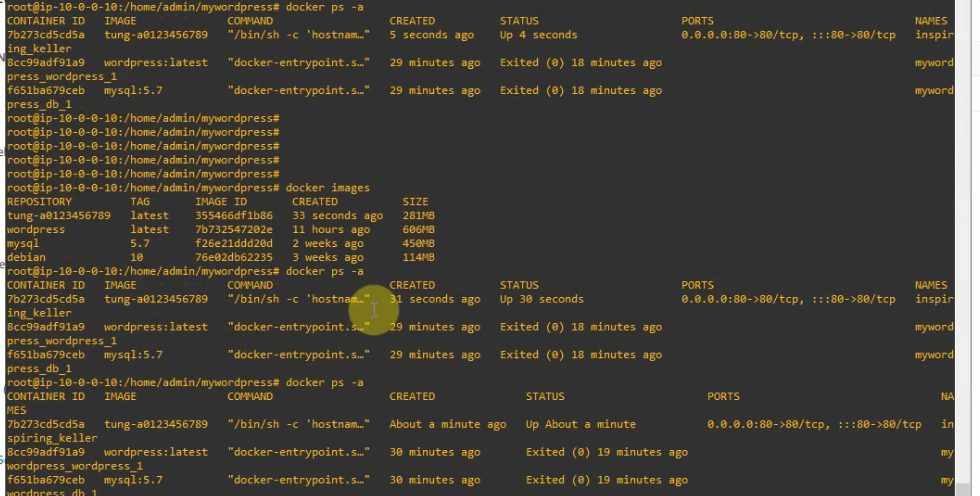