This is a diagram that I have used for this lab.
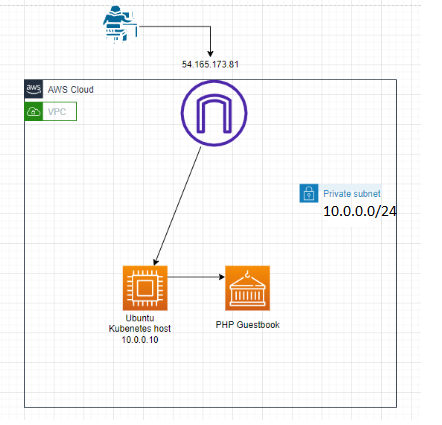
I have used the following link to deploy the PHP Guestbook application with Redis (https://kubernetes.io/docs/tutorials/stateless-application/guestbook/)
Set up an Ubuntu Linux with 2GB RAM and 30GB storage for the Kubernetes host. Allow SSH and HTTP from anywhere to the Linux instance on Security Group.
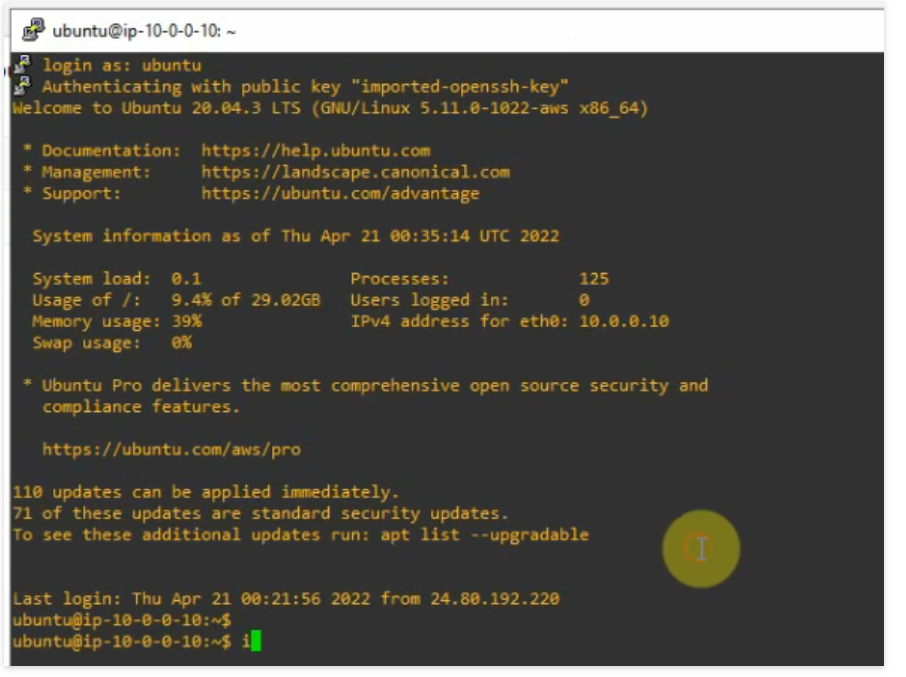
+ Deployment Redis Database.
Creating the Redis Deployment
#nano redis-leader-deployment.yaml
# SOURCE: https://cloud.google.com/kubernetes-engine/docs/tutorials/guestbook
apiVersion: apps/v1
kind: Deployment
metadata:
name: redis-leader
labels:
app: redis
role: leader
tier: backend
spec:
replicas: 1
selector:
matchLabels:
app: redis
template:
metadata:
labels:
app: redis
role: leader
tier: backend
spec:
containers:
- name: leader
image: "docker.io/redis:6.0.5"
resources:
requests:
cpu: 100m
memory: 100Mi
ports:
- containerPort: 6379
#Apply the Redis deployment.
microk8s kubectl apply -f https://k8s.io/examples/application/guestbook/redis-leader-deployment.yaml
# Check Redis Pod is running
microk8s kubectl get pods
# View logs from the Redis leader Pod
microk8s kubectl logs -f deployment/redis-leader
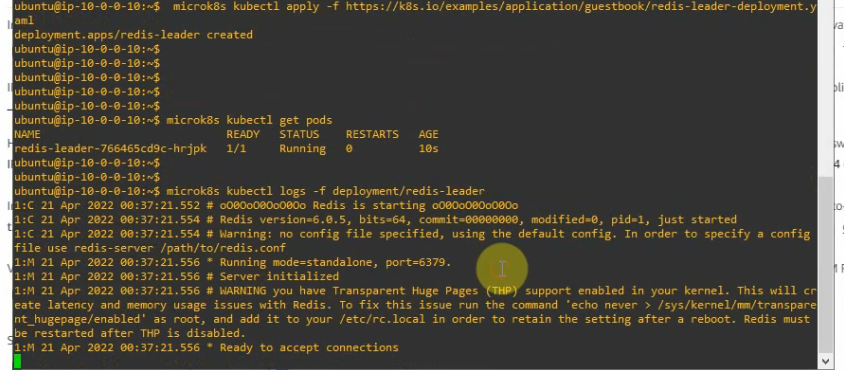
+ Creating the Redis leader Service
#Apply a service to proxy the traffic to the Redis Pod.
#nano redis-leader-service.yaml
# SOURCE: https://cloud.google.com/kubernetes-engine/docs/tutorials/guestbook
apiVersion: v1
kind: Service
metadata:
name: redis-leader
labels:
app: redis
role: leader
tier: backend
spec:
ports:
- port: 6379
targetPort: 6379
selector:
app: redis
role: leader
tier: backend
#Apply the Redis Service with the deployment file.
microk8s kubectl apply -f https://k8s.io/examples/application/guestbook/redis-leader-service.yaml
#verify that the Redis service is running
microk8s kubectl get service
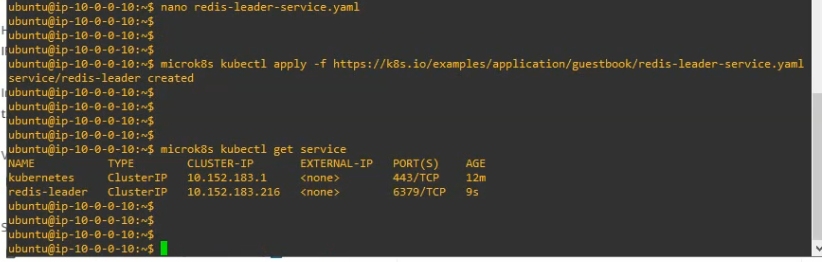
+ Set up Redis followers to make it highly available on a few Redis followers.
#nano redis-follower-deployment.yaml
# SOURCE: https://cloud.google.com/kubernetes-engine/docs/tutorials/guestbook
apiVersion: apps/v1
kind: Deployment
metadata:
name: redis-follower
labels:
app: redis
role: follower
tier: backend
spec:
replicas: 2
selector:
matchLabels:
app: redis
template:
metadata:
labels:
app: redis
role: follower
tier: backend
spec:
containers:
- name: follower
image: gcr.io/google_samples/gb-redis-follower:v2
resources:
requests:
cpu: 100m
memory: 100Mi
ports:
- containerPort: 6379
#Apply the Redis Deployment for the redis-follower-deployment.yaml file.
microk8s kubectl apply -f https://k8s.io/examples/application/guestbook/redis-follower-deployment.yaml
# Verify two Redis follower replicas are running.
microk8s kubectl get pods
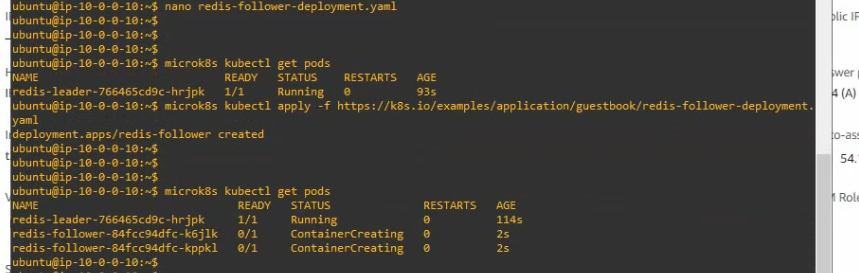
+ Creating the Redis follower service.
#nano redis-follower-service.yaml
# SOURCE: https://cloud.google.com/kubernetes-engine/docs/tutorials/guestbook
apiVersion: v1
kind: Service
metadata:
name: redis-follower
labels:
app: redis
role: follower
tier: backend
spec:
ports:
# the port that this service should serve on
- port: 6379
selector:
app: redis
role: follower
tier: backend
#Apply the Redis Service with the deployment file.
microk8s kubectl apply -f https://k8s.io/examples/application/guestbook/redis-follower-service.yaml
# verify that the Redis service is running.
microk8s kubectl get service
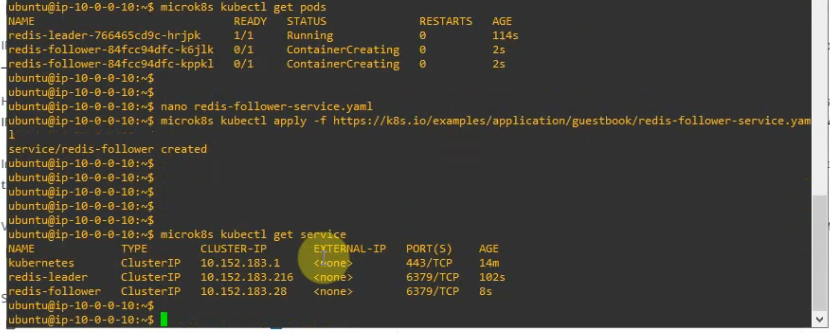
+ Set up and Expose the Guestbook Frontend.
#Creating Guestbook Frontend Deployment.
#nano frontend-deployment.yaml
# SOURCE: https://cloud.google.com/kubernetes-engine/docs/tutorials/guestbook
apiVersion: apps/v1
kind: Deployment
metadata:
name: frontend
spec:
replicas: 3
selector:
matchLabels:
app: guestbook
tier: frontend
template:
metadata:
labels:
app: guestbook
tier: frontend
spec:
containers:
- name: php-redis
image: gcr.io/google_samples/gb-frontend:v5
env:
- name: GET_HOSTS_FROM
value: "dns"
resources:
requests:
cpu: 100m
memory: 100Mi
ports:
- containerPort: 80
#Apply the frontend Deployment file
microk8s kubectl apply -f https://k8s.io/examples/application/guestbook/frontend-deployment.yaml
#Verify three frontend replicas are running.
microk8s kubectl get pods -l app=guestbook -l tier=frontend
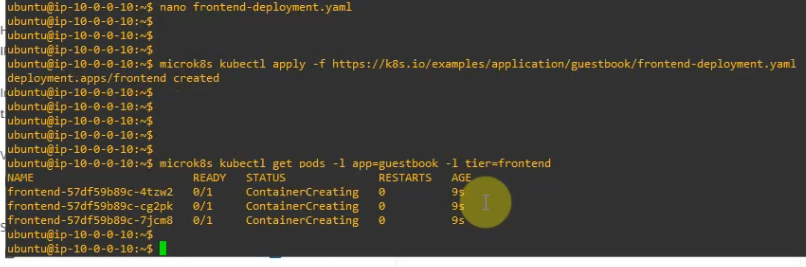
+ Creating the Frontend Service.
#nano frontend-service.yaml
# SOURCE: https://cloud.google.com/kubernetes-engine/docs/tutorials/guestbook
apiVersion: v1
kind: Service
metadata:
name: frontend
labels:
app: guestbook
tier: frontend
spec:
# if your cluster supports it, uncomment the following to automatically create
# an external load-balanced IP for the frontend service.
# type: LoadBalancer
#type: LoadBalancer
ports:
# the port that this service should serve on
- port: 80
selector:
app: guestbook
tier: frontend
#Apply the frontend Service with the deployment file.
microk8s kubectl apply -f https://k8s.io/examples/application/guestbook/frontend-service.yaml
#Verify three frontend replicas are running.
microk8s kubectl get services
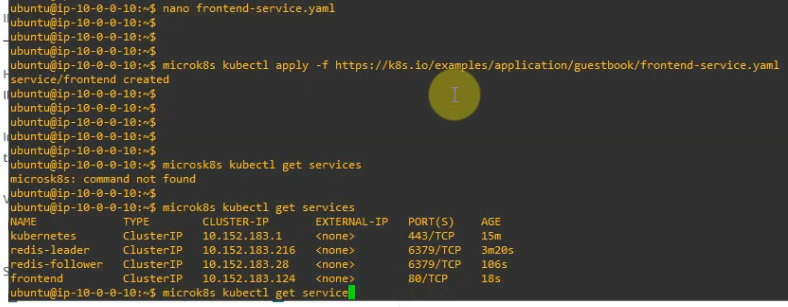
#Check the Frontend Service via LoadBalancer
microk8s kubectl get service frontend

+ Scale the Web Frontend.
microk8s kubectl scale deployment frontend --replicas=5
microk8s kubectl get pods
microk8s kubectl scale deployment frontend --replicas=2
microk8s kubectl get pods
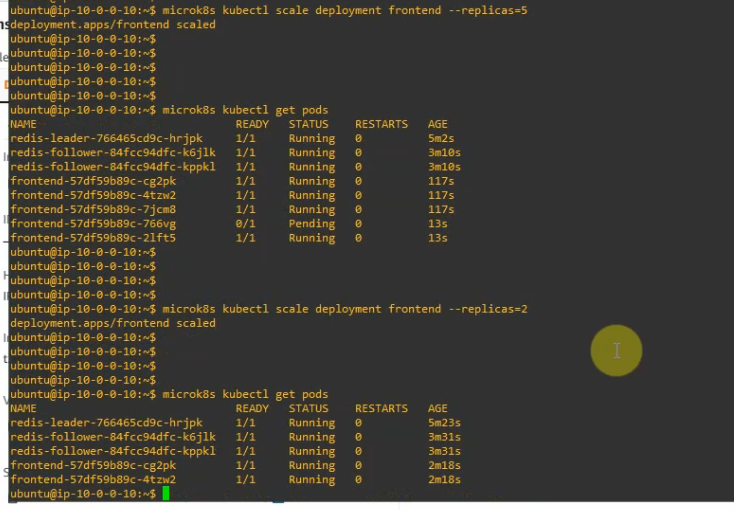
#check frontend service.

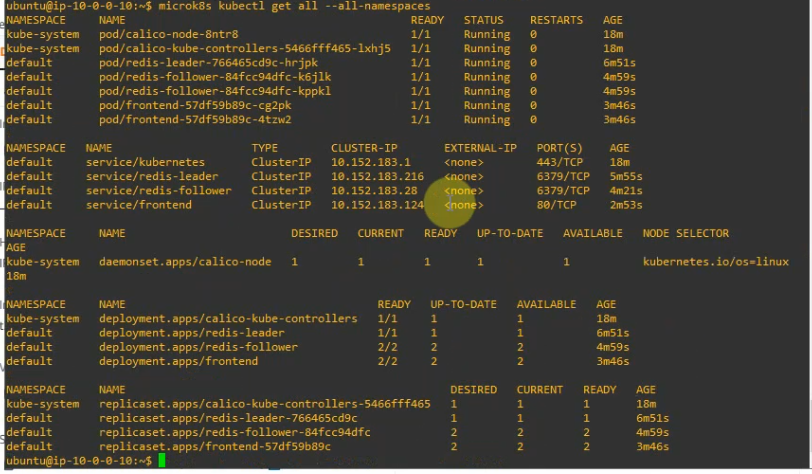
+ Expose port 80 via public IP address on Kubernetes host (10.0.0.10). This allows accessing PHP Guestbook from outside.
microk8s kubectl patch svc frontend -n default -p '{"spec": {"type": "LoadBalancer", "externalIPs":["10.0.0.10"]}}'

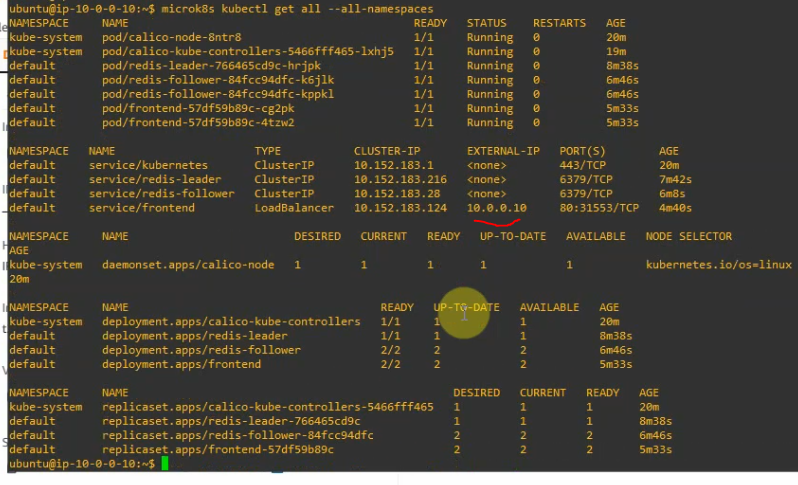

Access PHP Guestbook from the Internet (http://54.165.173.81).
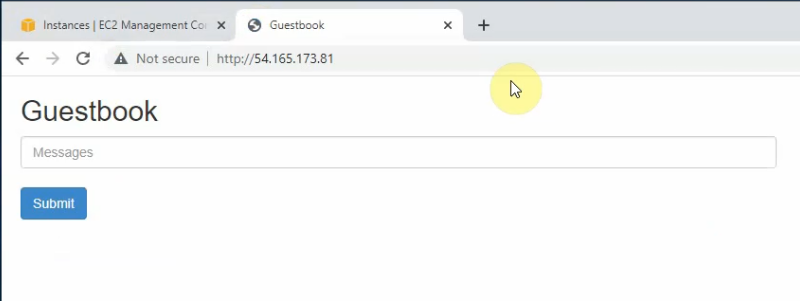