In this article, I will demonstrate how to count how many IP addresses in US-East-1 region.
We can get a list of IP address from AWS.
https://ip-ranges.amazonaws.com/ip-ranges.json
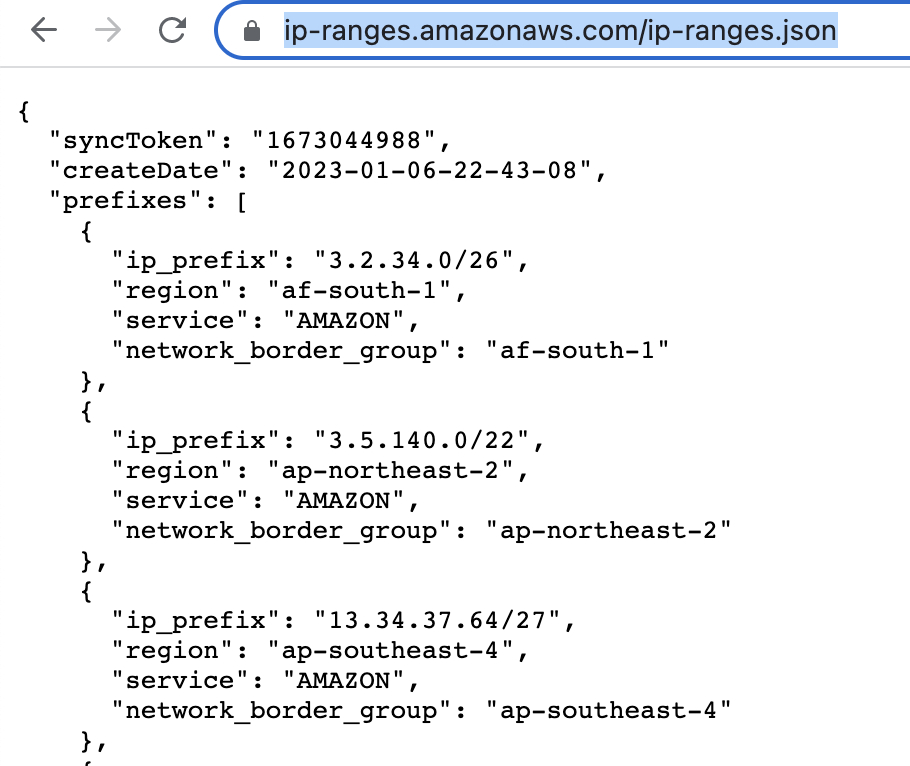
Download and save the JSON file to aws-ip-ranges.json in the present working directory.
Invoke-WebRequest -Uri https://ip-ranges.amazonaws.com/ip-ranges.json -OutFile aws-ip-ranges.json

Load the JSON file and convert JSON formatted file to custom PSObject of PowerShell.
$data = Get-Content -Path aws-ip-ranges.json -Raw | ConvertFrom-Json

Extract the list of IP addresses for the US-East-1 region and AMAZON service.
$ip_addresses = $data.prefixes | Where-Object { $_.region -eq 'us-east-1' -and $_.service -eq 'AMAZON' } | Select-Object -ExpandProperty ip_prefix
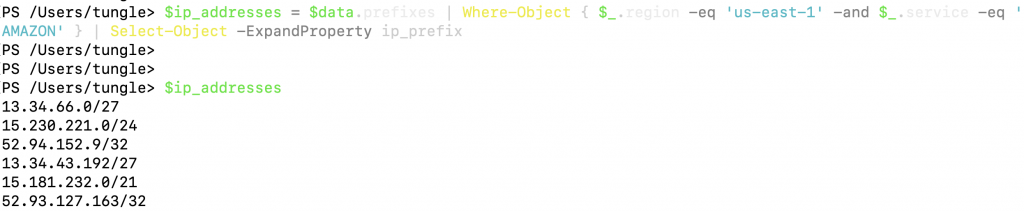
Below is a PS script.
# This script is using to extract IP address in US-East-1 region of AWS
# Download and save the JSON file to aws-ip-ranges.json in the present working directory
Invoke-WebRequest -Uri https://ip-ranges.amazonaws.com/ip-ranges.json -OutFile aws-ip-ranges.json
# Load the JSON file and convert JSON formatted file to custom PSObject or Hashtable object of PowerShell
$data = Get-Content -Path aws-ip-ranges.json -Raw | ConvertFrom-Json
# Extract the list of IP addresses for the US-East-1 region1 and service is AMAZON
$ip_addresses = $data.prefixes | Where-Object { $_.region -eq 'us-east-1' -and $_.service -eq 'AMAZON' } | Select-Object -ExpandProperty ip_prefix
# Print the list of IP addresses of AWS US-East-1 Region
$ip_addresses
Run the script.
./aws-ip.PS1
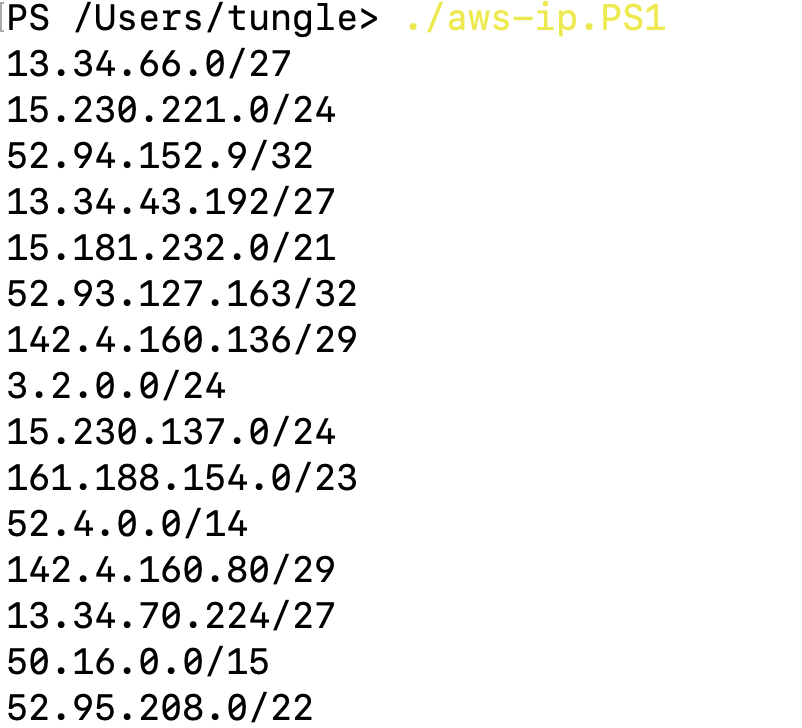
Save all range IP addresses when running the script aws-ip.PS1 to aws-ip.txt
./aws-ip.PS1 > aws-ip.txt
Using the following PowerShell script to extract how many IP addresses in the Amazon CIDR subnet such as 52.4.0.0/14.
param(
[string]$filename
)
function Count-IPs([string]$netblock) {
# Get CIDR value from the netblock
$cidr = [int]$netblock.Split('/')[1]
# Return the number of IP addresses in the netblock above
return [math]::Pow(2, 32 - $cidr)
}
if (!$filename) {
# Display the error message if the filename parameter is not provided
Write-Output "Usage: $($MyInvocation.MyCommand) <filename with CIDR masks>"
}
$ipcount = 0
Get-Content $filename | ForEach-Object {
$ipcount += Count-IPs $_
}
Write-Output $ipcount
Run the script.
./countips.PS1 aws-ip.txt

There are 17,728,227 IP addresses in AWS US-East-1 region.