ChatGPT is an AI chatbot system launched by OpenAI in November 2022. This is a great tool to help me understand more about PowerShell. Below are a couple of examples that I have used the tool to learn more PowerShell.
Can you show me top 10 commands in PowerShell?
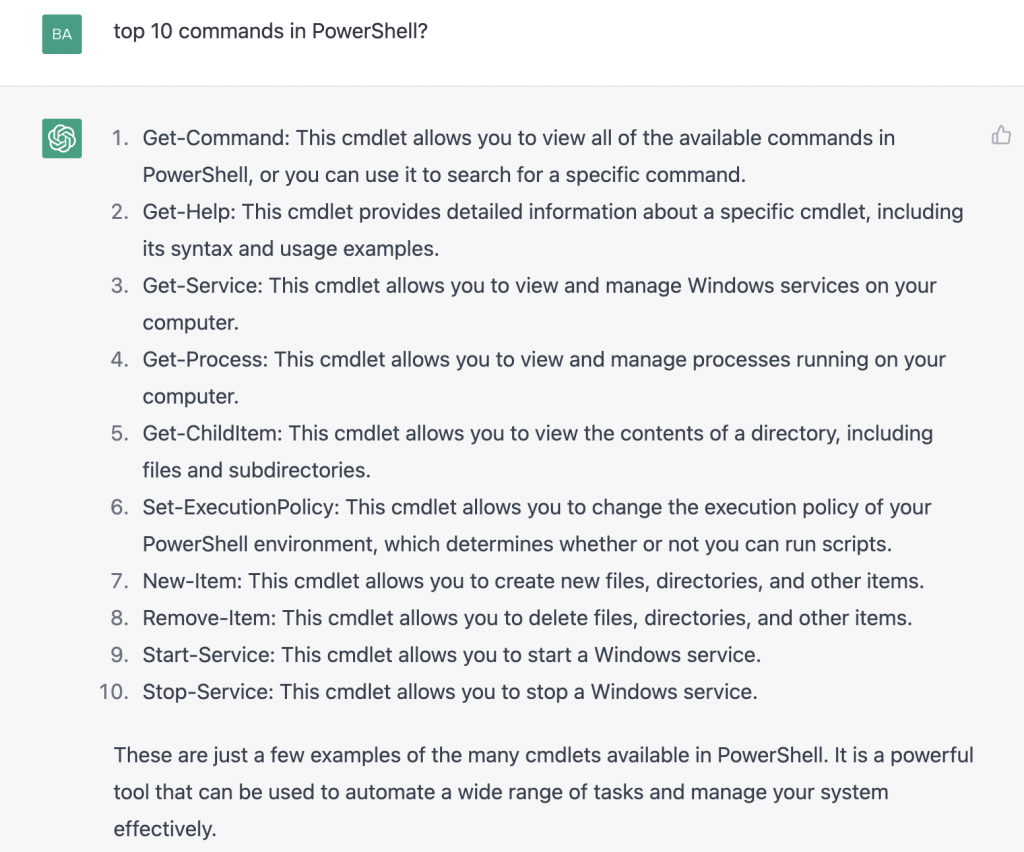
Can you rewrite the script below?
$Hosts = Get-Content "C:\Scripts\servers.txt"
$Ports = '443'
ForEach ($HOSTLINE in $hosts) {
$status = Test-NetConnection $HOSTLINE -Port $ports -WarningAction SilentlyContinue
if ($status.tcpTestSucceeded -eq $True){
Write-Output "$HOSTLINE is reachable"
}
else{
Write-Output "$HOSTLINE is unreachable"
}
}
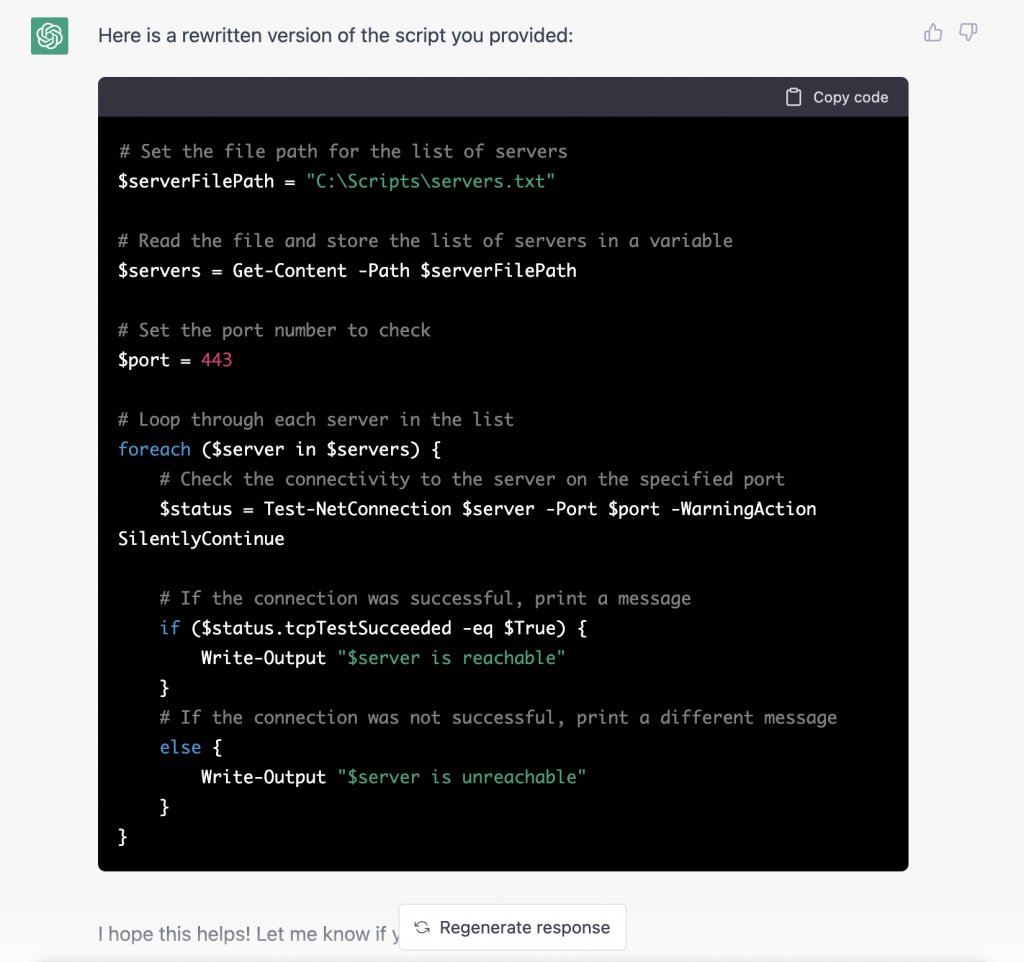
Tell me an example for using foreach-object parallel with PowerShell remoting?
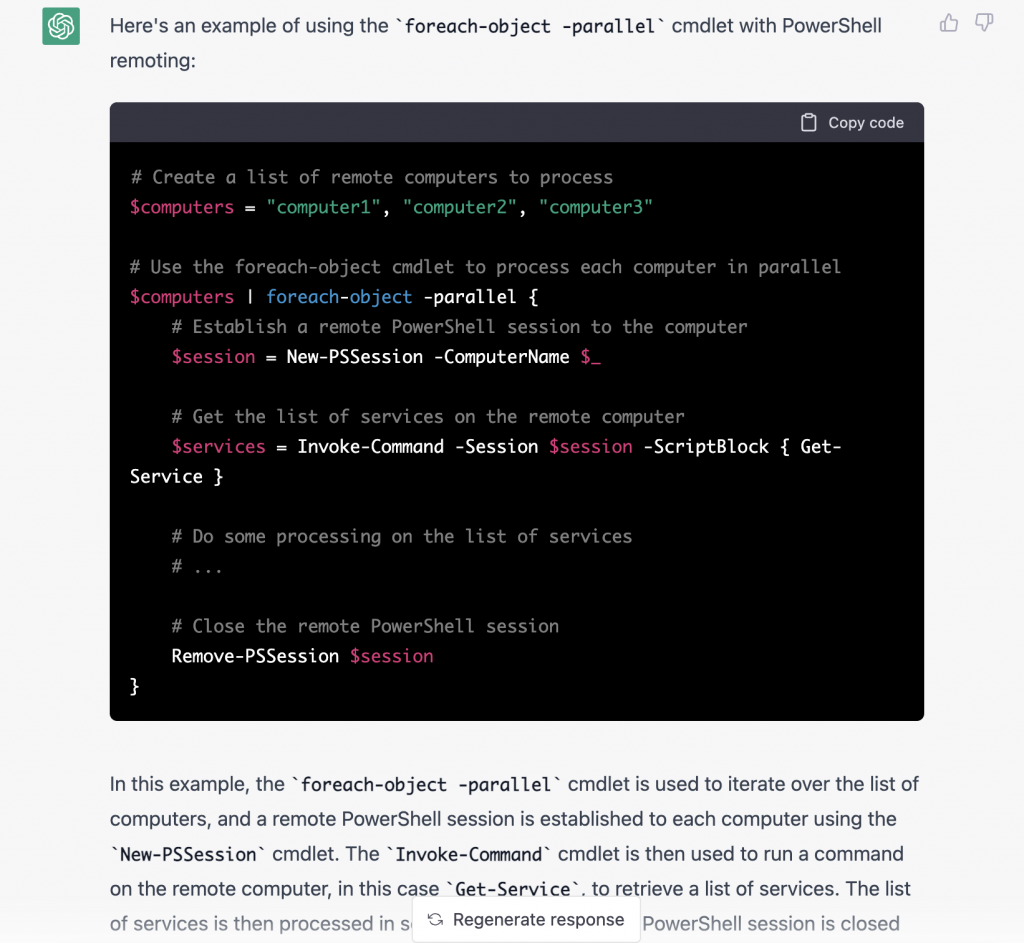
Does foreach-object parallel use multithreading?
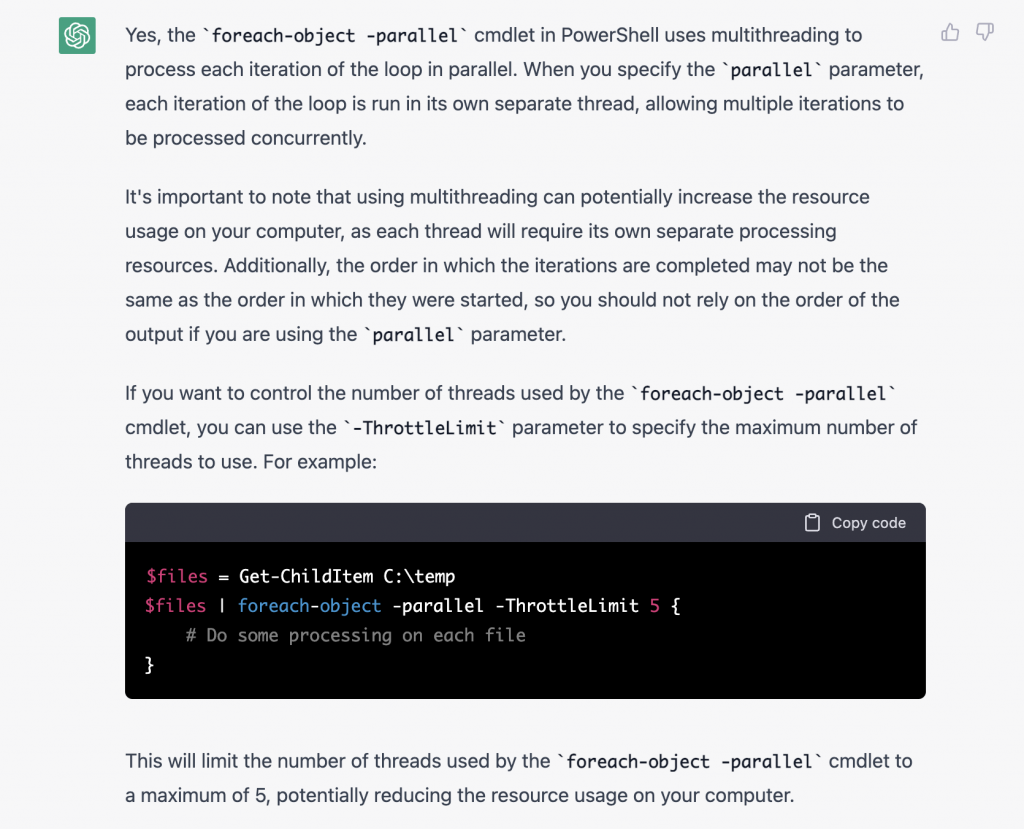
Is there a limitation of foreach-object parallel?
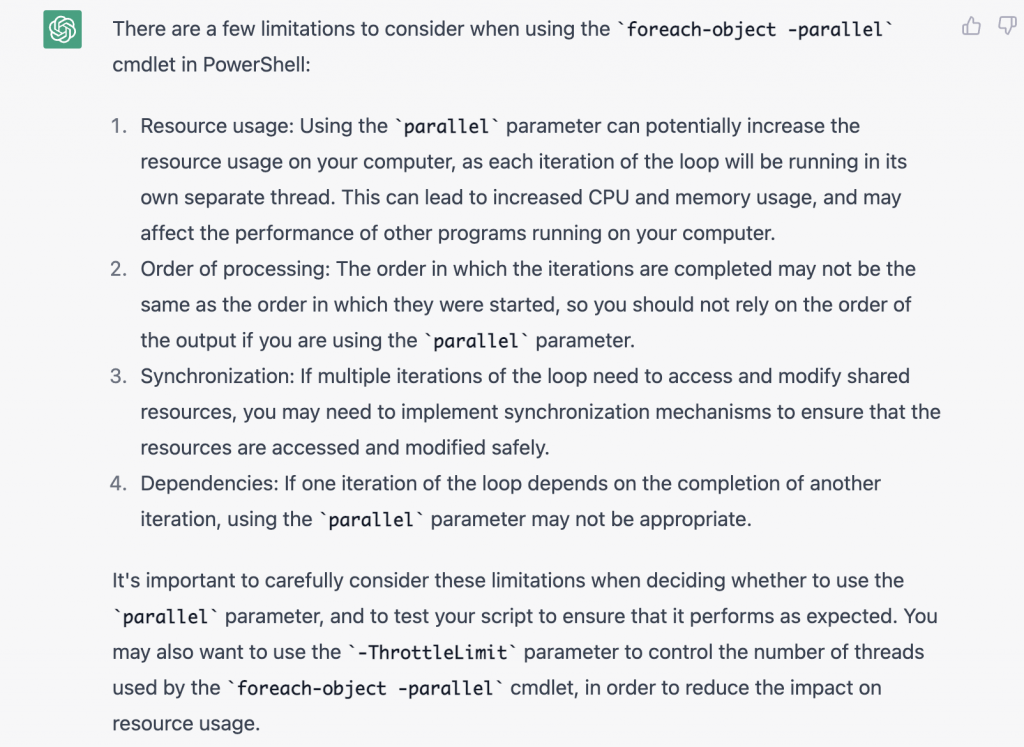
Show me an example to use foreach-object parallel to check CPU usage in remote machines?
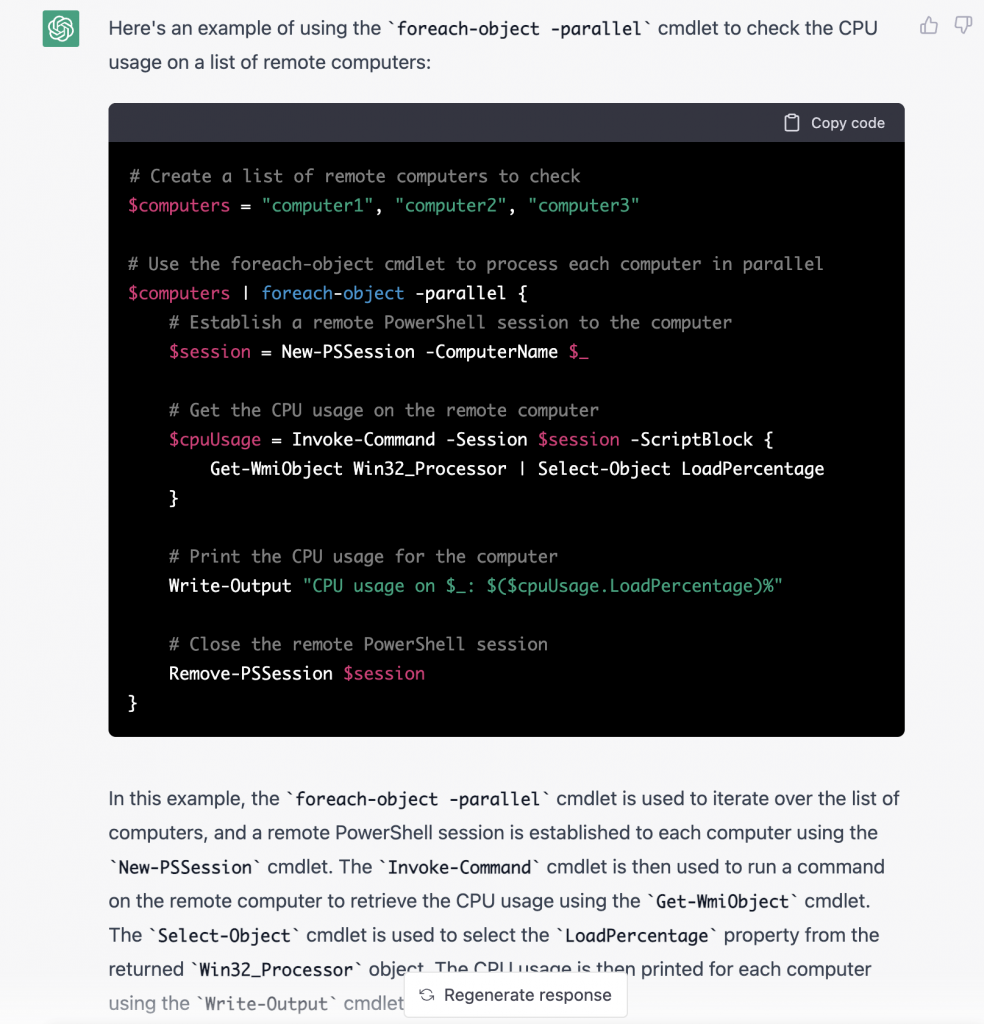
Can you rewrite the script below?
$inputpath = get-content "/var/log/auth.log"
#$inputpath = get-content "/Users/tungle/Downloads/auth.log.txt"
$regex = "\b\d{1,3}\.\d{1,3}\.\d{1,3}\.\d{1,3}\b"
$output = $inputpath | Select-String -Pattern $regex -AllMatches | Select-String "failed password for root" | % { $_.Matches } | % { $_.Value } `
| Group-Object | Select-Object Name, Count | Where-Object {$_.Count -gt 20}
ForEach($line in $output){
$IP = $line.Name
$result = Invoke-RestMethod -Method Get -Uri http://ip-api.com/json/$IP
# Sleep a second
Start-Sleep 1
#Write-Output $_count
$myObject = [PSCustomObject]@{
Count = $line.Count
"IPAddress" = $line.Name
#IP = $result.query
Country = $result.Country
}
$myObject
}
ChatGPT – Here is a rewritten version of the script you provided:
# Set the file path for the log file
$logFilePath = "/var/log/auth.log"
# Read the log file and store the contents in a variable
$logContent = Get-Content -Path $logFilePath
# Set the regular expression for extracting IP addresses
$ipRegex = "\b\d{1,3}\.\d{1,3}\.\d{1,3}\.\d{1,3}\b"
# Extract all of the IP addresses from the log file
$ipAddresses = $logContent | Select-String -Pattern $ipRegex -AllMatches | Select-Object -Expand Matches | Select-Object -Expand Value
# Group the IP addresses by count
$ipCounts = $ipAddresses | Group-Object | Select-Object Name, Count
# Filter the list to include only IP addresses that have failed login attempts more than 20 times
$filteredIpCounts = $ipCounts | Where-Object {$_.Count -gt 20}
# Loop through each IP address
foreach ($ipCount in $filteredIpCounts) {
# Get the IP address and the number of failed login attempts
$ip = $ipCount.Name
$count = $ipCount.Count
# Make a request to the IP address API to get information about the IP address
$ipInfo = Invoke-RestMethod -Method Get -Uri "http://ip-api.com/json/$ip"
# Sleep for a second to avoid making too many requests in a short period of time
Start-Sleep 1
# Create a custom object with the IP address, country, and number of failed login attempts
$result = [PSCustomObject]@{
"IPAddress" = $ip
Country = $ipInfo.Country
Count = $count
}
# Output the result
$result
}
The script was provided by ChatGPT did not work as my script. However, the script is clear and easier to understand. So, I only do a small change in the script to make it work.
PS /tmp> cat ./chatgpt.PS1
# Set the file path for the log file
$logFilePath = "/var/log/auth.log"
# Read the log file and store the contents in a variable
$logContent = Get-Content -Path $logFilePath
# Set the regular expression for extracting IP addresses
$ipRegex = "\b\d{1,3}\.\d{1,3}\.\d{1,3}\.\d{1,3}\b"
# Extract all of the IP addresses from the log file
$ipAddresses = $logContent | Select-String -Pattern $ipRegex -AllMatches | Select-String "failed password for root" | Select-Object -Expand Matches | Select-Object -Expand Value
# Group the IP addresses by count
$ipCounts = $ipAddresses | Group-Object | Select-Object Name, Count
# Filter the list to include only IP addresses that have failed login attempts more than 20 times
$filteredIpCounts = $ipCounts | Where-Object {$_.Count -gt 20}
# Loop through each IP address
foreach ($ipCount in $filteredIpCounts) {
# Get the IP address and the number of failed login attempts
$ip = $ipCount.Name
$count = $ipCount.Count
# Make a request to the IP address API to get information about the IP address
$ipInfo = Invoke-RestMethod -Method Get -Uri "http://ip-api.com/json/$ip"
# Sleep for a second to avoid making too many requests in a short period of time
Start-Sleep 1
# Create a custom object with the IP address, country, and number of failed login attempts
$result = [PSCustomObject]@{
"IPAddress" = $ip
Country = $ipInfo.Country
Count = $count
}
# Output the result
$result
}
./chatgpt.PS1 | Sort-Object Count -Descending
./show-attacker.PS1 | Sort-Object Count -Descending
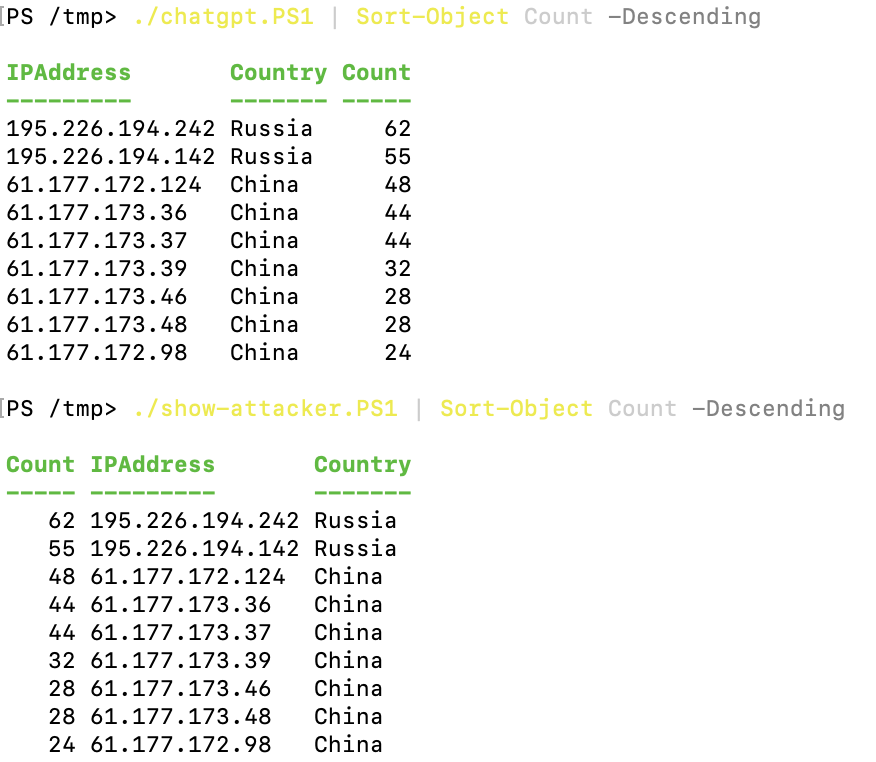