Below is a PS code that is used to query current OS build via PowerShell.
function Get-WindowsVersion{
[CmdletBinding()]
Param
(
[Parameter(Mandatory=$false,
ValueFromPipelineByPropertyName=$true,
ValueFromPipeline=$true
)]
[string[]]$ComputerName = $env:COMPUTERNAME
)
BEGIN {}
PROCESS {
Foreach ($Computer in $ComputerName)
{
try {
#$ping = Test-Connection -computerName $Computer -count 1 -Quiet
#$ping = Test-NetConnection -computerName $Computer -port 5985 -InformationAction SilentlyContinue
#if($ping)
#{
#$code = Get-ItemProperty -PATH 'HKLM:\SOFTWARE\Microsoft\Windows NT\CurrentVersion'
$Result = Invoke-Command -ComputerName $Computer -ScriptBlock {Get-ItemProperty -PATH 'HKLM:\SOFTWARE\Microsoft\Windows NT\CurrentVersion'} -ErrorAction Stop
$properties = [ordered]@{
ComputerName = $Computer
'Windows Edition' = $Result.ProductName
'Version' = $Result.ReleaseId
'OS Build' = $Result.CurrentBuild + '.' + $Result.UBR}
} catch {
#Write-Verbose "Coundn't connect to $computer"
$properties = [ordered]@{
ComputerName = $Computer
'Windows Edition' = $null
'Version' = $null
'OS Build' = $null
}
}
finally {
$obj = New-Object -Typename PSCustomObject -Property $properties
Write-Output $obj
}
}
}
}
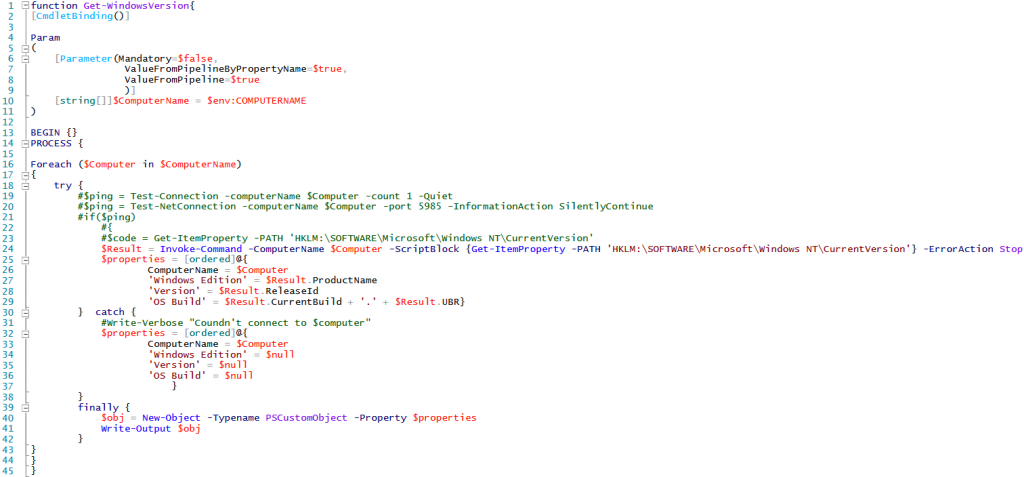
Get-WindowsVersion -computer W2016-S1
Get-WindowsVersion -ComputerName @('W2016-S1','DC3','DC1','DC2','Win10-PC') | sort Version -Descending
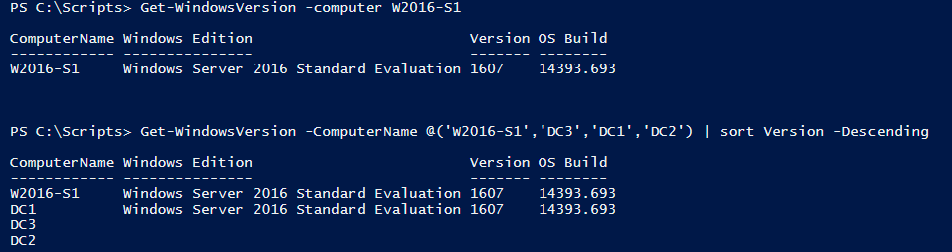
(DC2 and DC3 are not running or we cannot access to the servers for some reasons . So, we will create a hash table and assign null value for these computers.)